Thursday 27 August 2015
Saturday 22 August 2015
Matrix multiplication in C++
You have often learnt the method of matrix multiplication in Mathematics.
Matrix multiplication
Now,we will perform the same operations in C++ using the concept of 2-d arrays.So,below is the source code of the program.
Source code
#include<iostream>
#include<conio.h>
using namespace std;
void addition();
void subtraction();
void multiplication();
int mat1 [3][3], mat2[3][3],mat3[3][3], i ,j, k, sum,ab,ch;
int main()
{
int choice;
{
x:
cout<<"\t"<<"\t"<<"MAIN MENU"<<"\t"<<"\t"<<endl<<endl<<endl;
cout<<"Press 1 for 3*3 matrix multiplication."<<endl<<endl;
cout<<"Press 2 to exit from the program."<<endl<<endl;
cout<<"Enter your choice:";
cin>>choice;
switch(choice)
{
case 1:
multiplication();
break;
case 2:
return 0;
default:
cout<<"Invalid choice !!"<<endl<<endl;
}
goto x;
}
}
void multiplication()
{
cout<<"\nEnter values for first 3 x 3 matrix:\n";
for ( i = 0 ; i <= 2 ; i++ )
{
for (j = 0 ; j <= 2 ; j++ )
cin>>mat1 [i][j];
}
cout<<"\n Enter values for second 3 x 3 matrix:\n";
for ( i = 0 ; i <= 2 ; i++ )
{
for ( j = 0 ; j <= 2 ; j++ )
cin>>mat2[i][j];
}
cout<<"\n The first 3 x 3 matrix entered by you is:\n";
for ( i = 0 ; i <= 2 ; i++ )
{
for ( j = 0 ; j <= 2 ; j++ )
cout<< mat1[i][j]<<"\t";
cout<<endl<<endl;
}
cout<<"\n the second 3 x 3 matrix entered :\n";
for ( i = 0 ; i <= 2 ; i++ )
{
for ( j = 0 ; j <= 2 ; j++ )
cout<< mat2[i][j]<<"\t" ;
cout<<endl<<endl;
}
for ( i = 0 ; i <= 2 ; i++ )
{
for ( j = 0 ; j <= 2 ; j++ )
{
sum = 0;
for ( k = 0 ; k <=2 ; k++ )
sum = sum + mat1 [i][k] * mat2[k][j];
mat3[i][j] = sum ;
}
}
cout<<"\nThe product of the above two matrices is:\n";
for ( i = 0 ;i<= 2 ; i++ )
{
for ( j = 0 ; j <= 2 ; j++ )
cout<<mat3[i][j]<<"\t";
cout<<endl<<endl;
}
}
Output
Monday 17 August 2015
Matrix addition and subtraction in C++
You are well aware of the matrix addition and subtraction in Mathematics.
Matrix addition
Matrix subtraction
Now,we will perform the same operations in C++ using the concept of 2-d arrays.So,below is the source code of the program.
Source code
#include<iostream>
#include<conio.h>
using namespace std;
void addition();
void subtraction();
int mat1[2][2], mat2[2][2],mat3[2][2], i ,j, k, sum,ab,ch;
int main()
{
int choice;
{
x:
cout<<"\t"<<"\t"<<"MAIN MENU"<<"\t"<<"\t"<<endl<<endl<<endl;
cout<<"Press 1 for 2*2 matrix addition."<<endl<<endl;
cout<<"Press 2 for 2*2 matrix subtraction."<<endl<<endl;
cout<<"Press 3 to exit from the program."<<endl<<endl;
cout<<"Enter your choice:";
cin>>choice;
switch(choice)
{
case 1:
addition();
break;
case 2:
subtraction();
break;
case 3:
return 0;
default:
cout<<"Invalid choice !!"<<endl<<endl;
}
goto x;
}
}
void addition()
{
cout<<"\nEnter values for first 2 x 2 matrix:\n";
for ( i = 0 ; i <= 1 ; i++ )
{
for (j = 0 ; j <= 1 ; j++ )
cin>>mat1 [i][j];
}
cout<<"\n Enter values for second 2 x 2 matrix:\n";
for ( i = 0 ; i <= 1 ; i++ )
{
for ( j=0 ; j<=1 ; j++ )
cin>>mat2[i][j];
}
cout<<"\n The first 2 x 2 matrix entered by you is:\n";
for ( i=0 ; i<=1 ; i++ )
{
for ( j=0 ; j<=1 ; j++ )
cout<< mat1[i][j]<<"\t";
cout<<endl<<endl;
}
cout<<"\n the second 2 x 2 matrix entered :\n";
for ( i = 0 ; i <= 1 ; i++ )
{
for ( j = 0 ; j <= 1 ; j++ )
cout<< mat2[i][j]<<"\t";
cout<<endl<<endl;
}
for(i=0;i<=1;i++)
{
for(j=0;j<=1;j++)
{
mat3[i][j] = mat1[i][j] + mat2[i][j];
}
}
cout<<"The addition of matrices:"<<endl<<endl;
for(i=0;i<=1;i++)
{
for(j=0;j<=1;j++)
cout<<mat3[i][j]<<"\t";
cout<<endl<<endl;
}
}
void subtraction()
{
cout<<"\nEnter values for first 2 x 2 matrix:\n";
for ( i = 0 ; i <= 1 ; i++ )
{
for (j = 0 ; j <= 1 ; j++ )
cin>>mat1 [i][j];
}
cout<<"\n Enter values for second 3 x 3 matrix:\n";
for ( i = 0 ; i <= 1 ; i++ )
{
for ( j = 0 ; j <= 1 ; j++ )
cin>>mat2[i][j];
}
cout<<"\n The first 2 x 2 matrix entered by you is:\n"<<endl;
for ( i = 0 ; i <= 1 ; i++ )
{
for ( j = 0 ; j <= 1 ; j++ )
cout<< mat1[i][j]<<"\t";
cout<<endl<<endl;
}
cout<<"\n the second 2 x 2 matrix entered :\n"<<endl;
for ( i = 0 ; i <= 1 ; i++ )
{
for ( j = 0 ; j <= 1 ; j++ )
cout<< mat2[i][j]<<"\t";
cout<<endl<<endl;
}
for(i=0;i<=1;i++)
{
for(j=0;j<=1;j++)
mat3[i][j]=mat1[i][j]-mat2[i][j];
}
cout<<"The subtraction of matrices:"<<endl<<endl;
for(i=0;i<=1;i++)
{
for(j=0;j<=1;j++)
cout<<mat3[i][j]<<"\t";
cout<<endl<<endl;
}
}
Output
Saturday 15 August 2015
Initialization of 2-d arrays in C++
Initializing 2-dimensional array
The 2-D arrays which are declared by the keyword int and able to store integer values are called two dimensional integer arrays. The process of assigning values during declaration is called initialization. TheseArrays can be initialized by putting the curly braces around each row separating by a comma also each element of a matrix should be separated by a comma.
Example:
int mat[3][3]={ {2,3},{4,5},{6,7} }
Initializing 2-d character array
The 2-D arrays which are declared by the keyword char and able to store characters are called two dimensional character arrays. 2-D character array can be initialized by putting the curly braces around each row as well as apostrophe around every alphabet.
Example:
char character[3][3]={'b', 'a' ,'t' ,'f', 'a' ,'t' ,'m' ,'a' ,'t'};
reference
The reference of this article is taken from
In my next blog inshAllah,I will post an important example of 2-d arrays that will be very helpful in understanding this concept.So,stay tuned.
Labels:
Arrays,
C,
C++,
C++ coding,
C++ programming,
C++ programs,
Coding,
CPP,
Programs
Thursday 13 August 2015
C++ The Complete Reference
Introduction
C++ The Complete Reference
Master programmer and best-selling author Herb Schildt has updated and expanded his classic reference to C++. Using expertly crafted explanations, insider tips, and hundreds of examples, Schildt explains and demonstrates every aspect of C++. Inside you'll find details on the entire C++ language, including its keywords, operators, preprocessor directives, and libraries. There is even a synopsis of the extended keywords used for .NET programming. Of course, everything is presented in the clear, crisp, uncompromising style that has made Herb Schildt the choice of millions. Whether you're a beginning programmer or a seasoned pro, the answers to all your C++ questions can be found in this lasting resource.
Detailed coverage includes:
- Data types and operators
- Control statements
- Functions
- Classes and objects
- Constructors and destructors
- Function and operator overloading
- Inheritance
- Virtual functions
- Namespaces
- Templates
- Exception handling
- The I/O library
- The Standard Template Library (STL)
- Containers, algorithms, and iterators
- Principles of object-oriented programming (OOP)
- Runtime type ID (RTTI)
- The preprocessor
- Much, much more
Author:
Herbert Schildt
Date of publishing:
November 19, 2002
Publisher:
McGraw-Hill Education
Language:
English
Genre:
Programming
ISBN:
- ISBN-10: 0072226803
- ISBN-13: 978-0072226805
Rating:
4.3/5.0 stars
Votes:
37
Labels:
books,
C,
C++,
C++ books,
computer,
CPP,
khgamujtaba,
programming
Wednesday 12 August 2015
Two-dimensional arrays in C++
Definition
In C++ Two Dimensional array in C++ is an array that consists of more than one rows and more than one column. In 2-D array each element is refer by two indexes. Elements stored in these Arrays in the form of matrices. The first index shows a row of the matrix and the second index shows the column of the matrix.
Syntax
data type name of array[no. of rows][no. of columns]
For example,
int item[4][5];
Accessing each location
In order to store values in a C++ two dimensional arrays the programmer have to specified the number of row and the number of column of a matrix. To access each individual location of a matrix to store the values the user have to provide exact number of row and number of column.
Example:
int matrix[2][2];
matrix[0][0]=20;
matrix[0][1]=25;
matrix[1][0]=30;
matrix[1][1]=35;
Entering data
Nested loop is used to enter data in 2-D arrays. It depends upon the programmer which loop he wants to use it could be While loop or it could be a For loop. The outer loop acts as the number of rows of a matrix and the inner loop acts as the number of columns of a matrix.
The reference of this article has been taken from
Example:
#include<iostream.h> #include<conio.h> main() { int matrix [5] [5]; for ( int m1=0 ; m1<5 ; m1++) { for ( int m2=0 ; m2<5 ; m2++) { Matrix [m1] [m2] = 5 ; } } getch(); }
|
Tuesday 11 August 2015
Monday 10 August 2015
Boorland for Windows
Introduction
The Borland C++ 5.5 Compiler is the high performance foundation and core technology of Inprise/Borland's award-winning Borland C++Builder product line and is the basis for Inprise/Borland's recently announced C++Builder(TM) 5 development system for Windows 95, 98, NT, and Windows 2000.
"Over the past 11 years, millions of developers have relied on the speed and quality of the Borland C/C++ compiler technology. Beginning this month, every developer will have free access to the latest Borland C/C++ compiler for building high quality Windows, Internet, and distributed applications," said Michael Swindell, director of product management at Inprise/Borland. "With Open Source development exploding on all platforms, developers can now rely on a leading commercial ANSI C++ compiler to be available for any Windows based Open Source project. In addition, with our forthcoming Linux C++ tools, development with Borland C++ tools today is an investment in Linux development for tomorrow."
"Since many programmers learned how to develop using Borland tools, it's great to see Inprise/Borland offer its widely-used compiler free of charge," said Sally Cusack, an analyst and research manager at International Data Corporation. "Developers who download this compiler will subsequently have a seamless path to the rich tools and capabilities of Borland C++Builder 5 for RAD, Internet, User Interface, database, and distributed solutions."
Price:
0.0 $
Official URL:
Download URL:
Rating:
4/5 stars
Reviewer:
Reviewer date:
04/12/2011
Sunday 9 August 2015
Swapping two numbers
"Swapping two number" is one of the most popular and easiest program of C++. If you want to make a good logic in C++,then you should practice this code.
Today I am here with the source code of this program.Hope you will like my effort.
Today I am here with the source code of this program.Hope you will like my effort.
Source code
Output
Logic
Below is the logic of this program.
I have taken 3 variables for this program,"a,b and tem(or temporary).
According to logic,
Suppose the value of a is 10 and the value of b is 20.
According to the given condition,
tem=a;
tem=10;
a=b
10=b or b=10;
b=tem
20=tem
b=10.
So,after swapping,the value of "a" becomes 20 and the value of "b" becomes 10.
The ANCI C programming language
INTRODUCTION
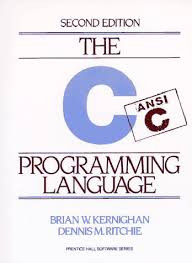
The ANCI C programming language
The authors present the complete guide to ANSI standard C language programming. Written by the developers of C, this new version helps readers keep up with the finalized ANSI standard for C while showing how to take advantage of C's rich set of operators, economy of expression, improved control flow, and data structures. The 2/E has been completely rewritten with additional examples and problem sets to clarify the implementation of difficult language constructs. For years, C programmers have let K&R guide them to building well-structured and efficient programs. Now this same help is available to those working with ANSI compilers. Includes detailed coverage of the C language plus the official C language reference manual for at-a-glance help with syntax notation, declarations, ANSI changes, scope rules, and the list goes on and on.
Authors:
Brian W.Kernighan and Dennis.M.Ritchie.
Date of publishing:
April 1,1988.
Publisher:
Prentice Hall.
Language:
English
genre:
programming.
ISBN:
ISBN-10: 0131103628
- ISBN-13: 978-0131103627
Rating:
4.5/5.0 stars
Votes:
514
Reviewer:
Mark Cristie
![]() |
The ANCI C programming language |
Authors:
Brian W.Kernighan and Dennis.M.Ritchie.
Date of publishing:
April 1,1988.
Publisher:
Prentice Hall.
Language:
English
genre:
programming.
ISBN-10: 0131103628
- ISBN-13: 978-0131103627
Rating:
4.5/5.0 stars
Votes:
514
Reviewer:
Mark Cristie
Mark Cristie
Saturday 8 August 2015
Arrays in C++
Concept of arrays
One of the most important concepts in C++ is arrays.Today I will explain the concept of arrays with daily life examples.For this article,I am taking help from the Android application "C++ little drops".
Suppose we want to calculate the average of the marks for a class of 20 students ,we certainly do not want to create 30 variables: std1,st2,std3,.......std.20.Instead,we can use a single variable,called an array with 30 elements.
Definition of arrays
An array is a list of elements of the same type(e.g., int), identified by a pair of square brackets [ ].
Declaration of arrays
To use an array, we need to declare the array with 3 things: a name, a type and a dimension( or size, or length).Remember that an array always starts with index 0.It means that the first value in an array is stored in index 0.The syntax is as follows:
Syntax
type arrayName[array length];
It is recommended to use a plural name for array.e.g., marks,numbers, books,etc.
int books[10];
We can also initialize the array during declaration with a comma-separated list of values,as follows:
int numbers{2,4,5,6,3,9,12,14};
Example
A simple program will help you understand this concept more clearly.
Source code
#include<iostream>
#include<conio.h>
using namespace std;
main()
{
int numbers[5]={1,2,3,4,5}; //an array "numbers"
cout<<"The first number: "<<numbers[0]<<endl; // number stored in index 0;
cout<<"The second number: "<<numbers[1]<<endl;
cout<<"The third number: "<<numbers[2]<<endl;
cout<<"The fourth number: "<<numbers[3]<<endl;
cout<<"The fifth number:"<<numbers[4]<<endl;
}
Output
Friday 7 August 2015
Tuesday 4 August 2015
Subscribe to:
Posts (Atom)